Validating email addresses is a common requirement in many Python applications. Whether you are building a form validation feature or need to ensure the accuracy of user input, implementing proper email validation is essential. Python provides several methods and libraries that simplify the process of email checking. In this comprehensive guide, we will explore various approaches to validate email addresses in Python. We will cover regular expressions, built-in Python functions, and popular libraries that streamline email validation. By the end of this article, you will have a solid understanding of how to check email addresses in Python and ensure the integrity of your data.
The Importance of Email Address Validation
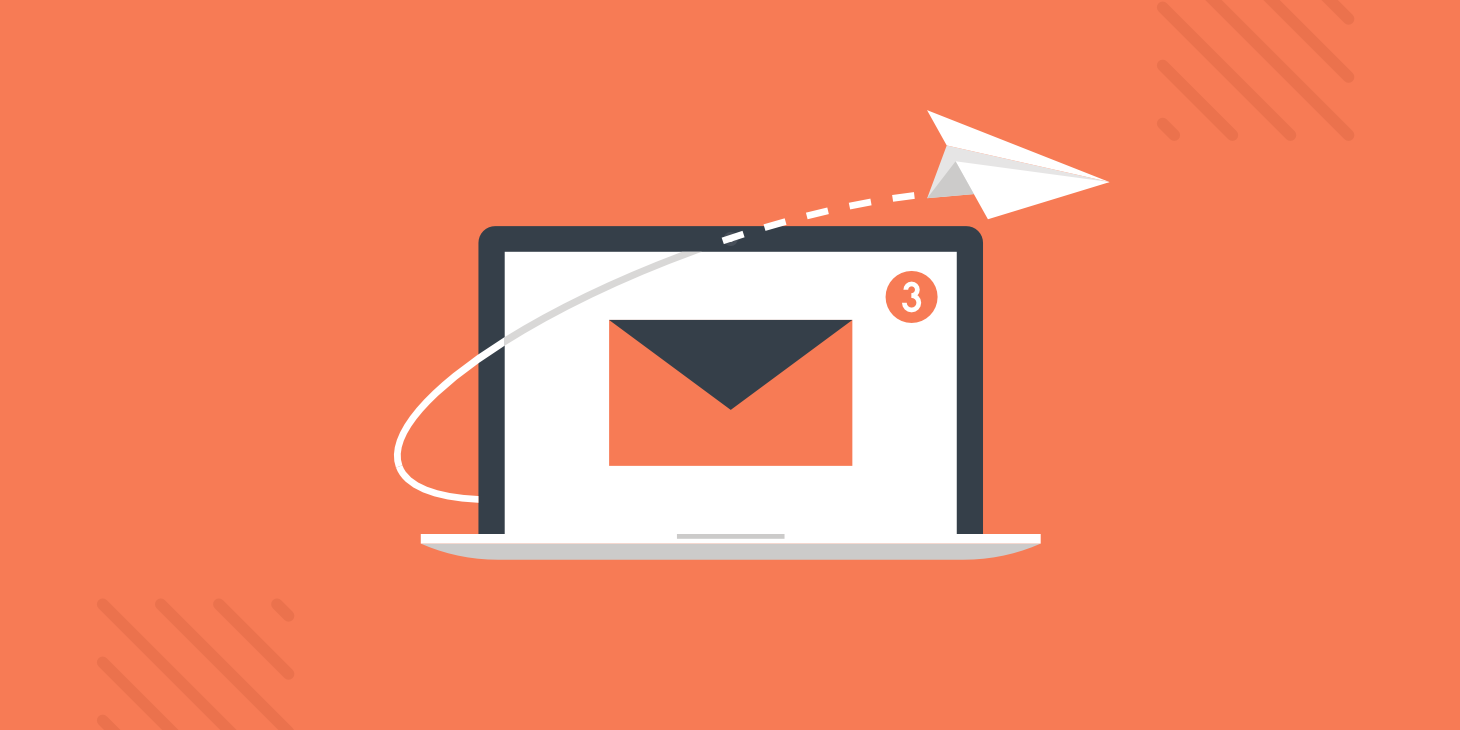
Email address validation is crucial for maintaining data accuracy, improving user experience, and ensuring the proper functioning of your applications. By implementing email validation, you can achieve the following benefits:
1. Data Accuracy
Validating email addresses helps ensure that the addresses provided by users are in the correct format. By validating the input, you can prevent invalid or malformed email addresses from being stored in your database or used for communication purposes.
2. User Experience
Real-time email address validation during form submission enhances the user experience. By providing immediate feedback on the validity of email addresses, you can guide users to correct any errors and prevent submission of incorrect data.
3. Data Integrity
Validating email addresses protects the integrity of your data. By verifying the format and structure of email addresses, you can prevent potential issues such as data corruption, incorrect data analysis, and compromised system functionality.
Approaches to Email Address Validation in Python
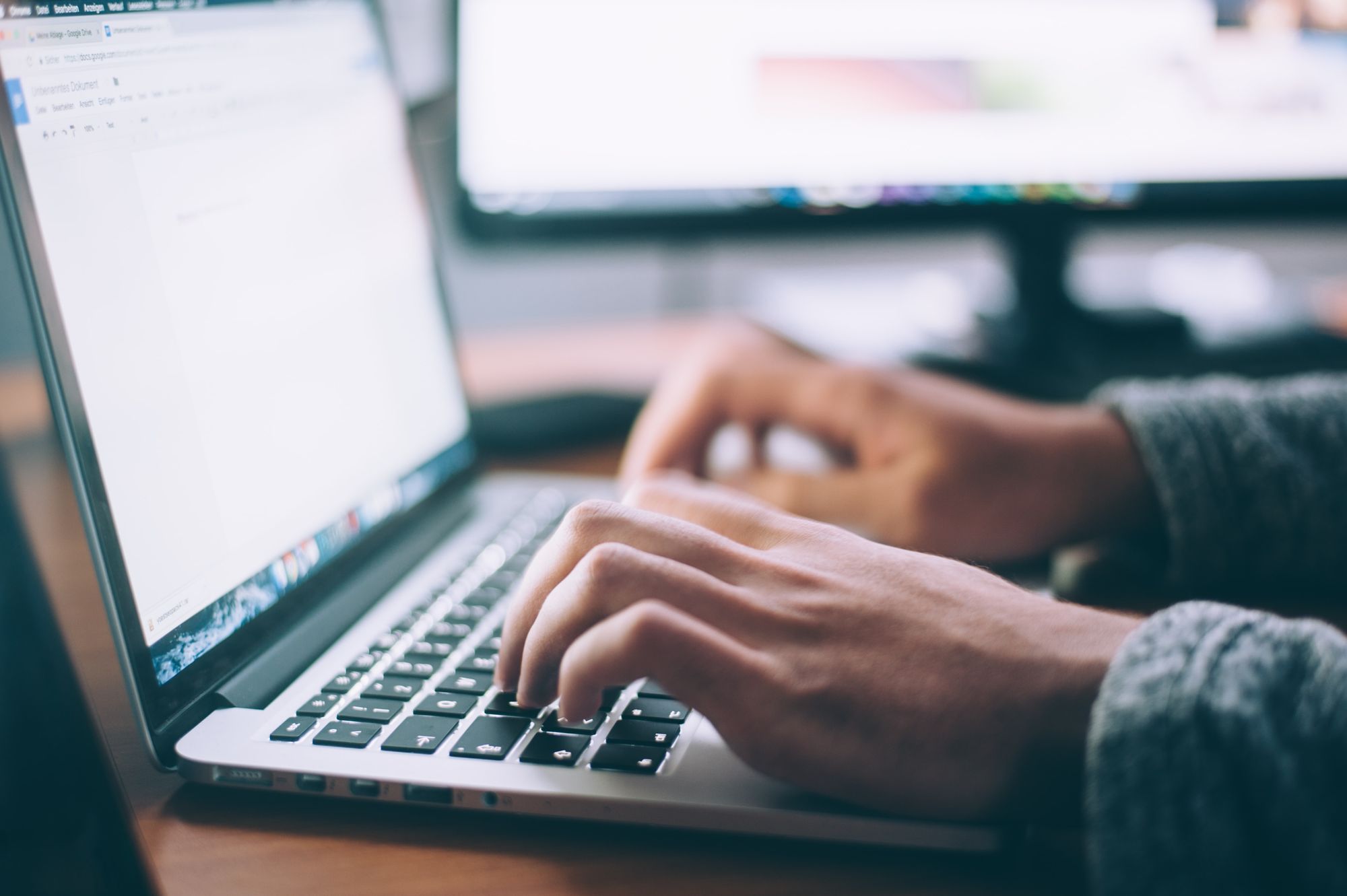
Python offers multiple approaches to validate email addresses. Let's explore some commonly used methods:
1. Regular Expressions
Regular expressions (regex) are powerful tools for pattern matching and validation. Python's built-in 're' module provides functions to match and validate email addresses using regex patterns. Here's an example of a simple regex pattern for email validation in Python:
import re
In this example, the 'validate_email()' function uses the 're.match()' function to check if the email matches the regex pattern. If the match is not None, the email is considered valid.
2. Built-in Python Functions
Python provides built-in functions and methods that can be used to validate email addresses. For example, the 'split()' method can be used to split the email address into two parts: the username and the domain. You can then perform specific checks on each part to validate the email.
def validate_email(email):
username, domain = email.split('@')
if len(username) == 0 or len(domain) == 0:
return False
return True
In this example, the 'validate_email()' function checks if the username and domain are not empty, indicating a valid email address.
3. External Libraries
Several Python libraries offer convenient methods and additional features for email address validation. Some popular libraries include:
- Email-Validator:
Email-Validator is a comprehensive library that provides various validation options, including syntax checking, domain existence verification, and MX record validation. It offers a simple and intuitive API for validating email addresses in Python.
- validate-email-address:
The validate-email-address library is specifically designed for email address validation. It provides functions to check the syntax, domain, and mailbox existence of an email address. It also offers options to perform DNS validation and additional checks.
- Py3DNS:
Py3DNS is a library that integrates with DNS resolvers to validate email addresses. It performs DNS lookups to check the existence of the domain and the validity of the mailbox server.
Implementing Email Address Validation in Python
Let's walk through an example of implementing email address validation in Python using regular expressions:
import re
def validate_email(email):
pattern = r'[2]+@[\w.-]+.\w+$'
return re.match(pattern, email) is not None
List of email addresses
email_list = ['[email protected]', '[email protected]', 'invalid_email']
Validate each email address
In this example, we define the 'validate_email()' function using the regex pattern to validate an individual email address. We iterate through each email address in the list and use the function to check its validity.
Conclusion
Email address validation is a critical aspect of ensuring data accuracy and improving user experience in Python applications. By using regular expressions, built-in Python functions, or external libraries, you can easily implement email address validation in your projects. Regular expressions offer flexibility and control, while libraries provide additional features and convenience. Choose the approach that best fits your requirements and apply it to validate email addresses effectively. By implementing proper email validation, you can enhance the reliability and usability of your applications and maintain the integrity of your data.