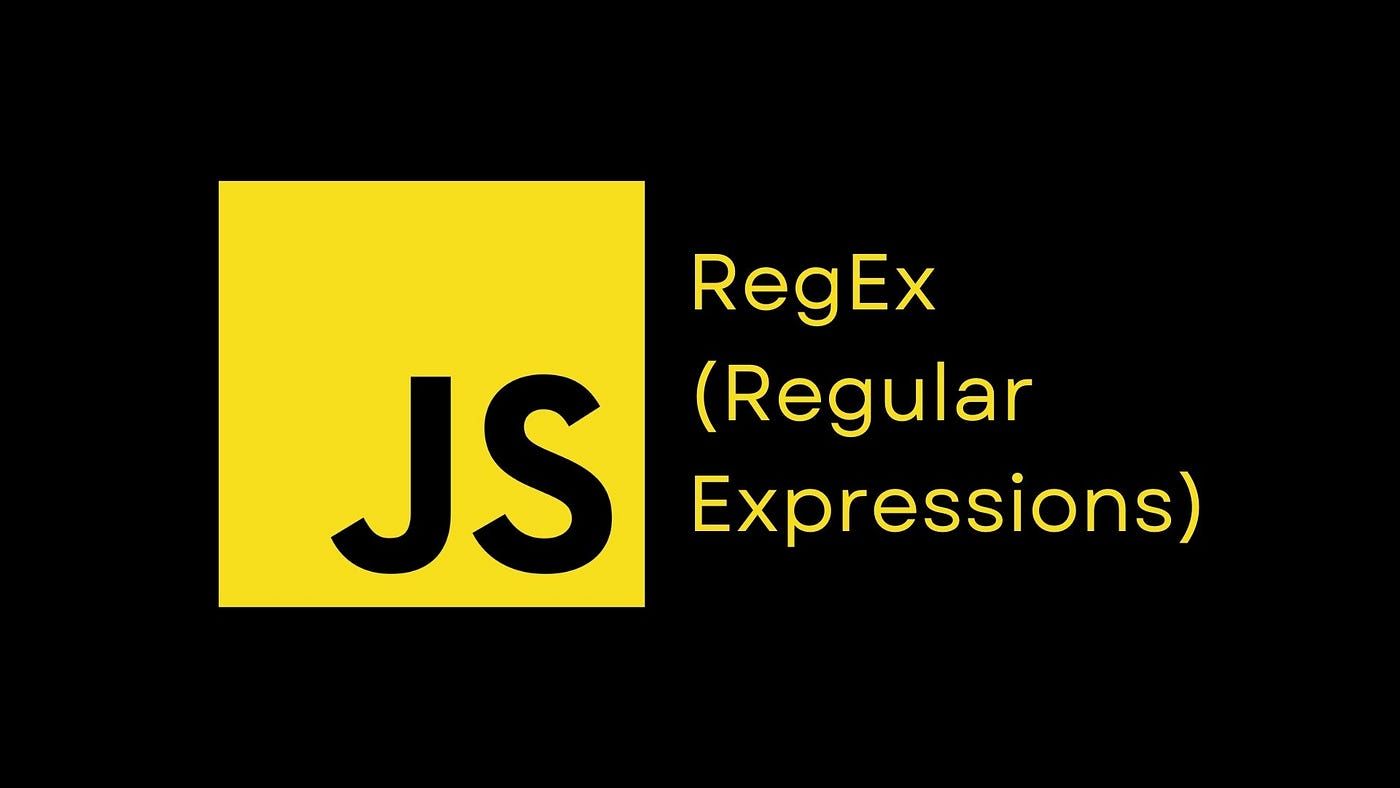
Email validation is a critical aspect of web development, ensuring that user-submitted email addresses are accurate and correctly formatted. In this comprehensive guide, we will explore the world of email validation in JavaScript using regular expressions (regex). You will become an expert in crafting and implementing email regex patterns, understand best practices, and learn how to overcome common validation issues. Whether you're a beginner or a seasoned developer, this guide will empower you to master email validation.
The Importance of Email Validation in JavaScript
Email validation in JavaScript offers several key benefits:
Data Quality: Accurate email validation ensures that the data collected from users is reliable and conforms to the expected email format.
User Experience: Validating email addresses enhances the user experience by preventing incorrect submissions.
Security: Proper email validation is crucial for user authentication and communication, reducing security risks.
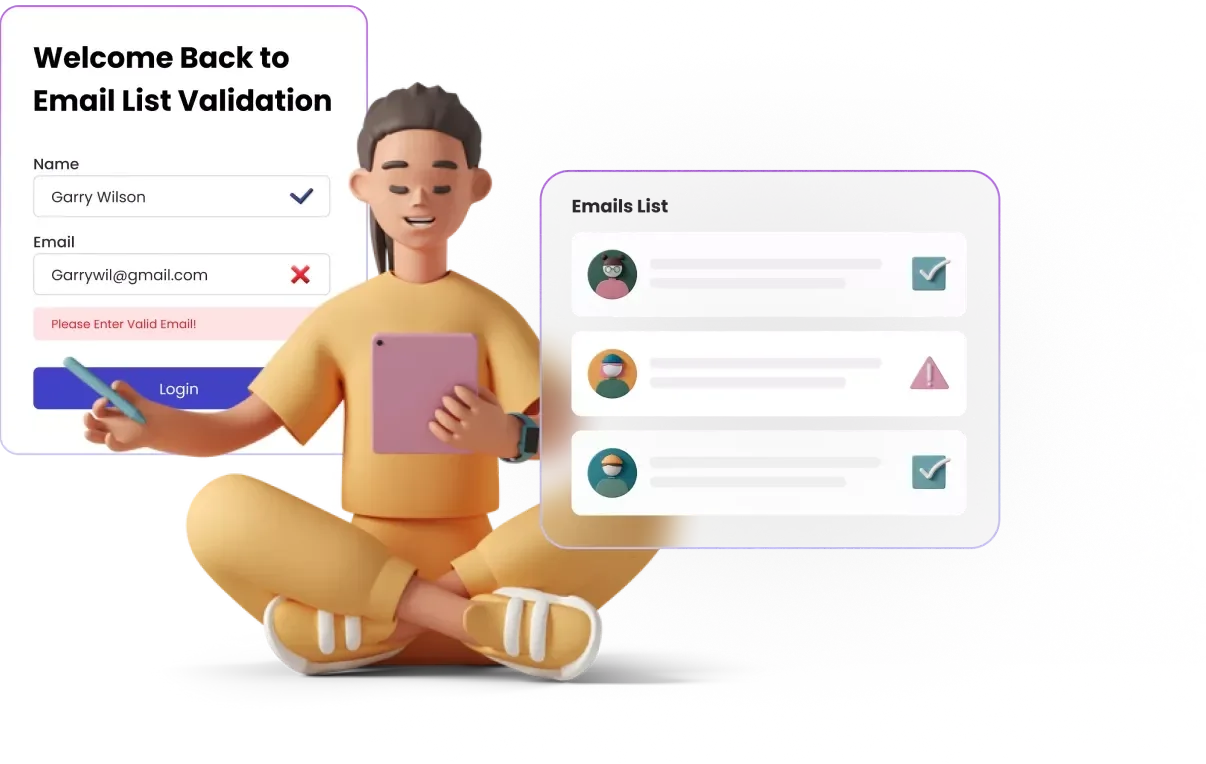
Enhancing your JavaScript email validation with the Email List Validation Single Verification API adds a superior layer of precision and trustworthiness to your process. This API offers real-time validation that goes well beyond simple regex checks, diving into the existence of email addresses, domain authenticity, and screening for temporary email services and catch-all domains. By weaving this API into your web development projects, you craft a more thorough and fail-safe email validation system. This upgrade not only boosts the reliability of the data you collect but also elevates the user experience by minimizing the likelihood of email delivery issues. Utilizing the API is straightforward: initiate an API call with the email in question, and receive a comprehensive validation report in return. This smooth integration guarantees that your digital platforms uphold the highest data integrity and security standards.
Our API employs key-based authentication for enhanced security. Retrieve your unique API key by navigating to the API section within your dashboard.
Email List Validation API
Email List Validation offers a versatile developer API, enabling seamless integration of our email verification service with your applications. Our API supports individual email checks as well as bulk verification through file uploads.
Verify Email Functionality
Submit email addresses for meticulous verification. We support various API libraries for ease of integration:
- Python: https://github.com/Xavierluijer/Emaillistvalidation-Python
- Ruby: https://github.com/Xavierluijer/Emaillistvalidation-Ruby
- PHP: https://github.com/Xavierluijer/Emaillistvalidation-PHP
- C#: https://github.com/Xavierluijer/Emaillistvalidation-CSharp
Verification responses are conveyed as straightforward text strings, indicating:
- ok: The email has cleared all verification checks.
- fail: The email failed one or several checks.
- unknown: The email's validity couldn't be definitively determined.
- incorrect: No email was provided, or it contains syntax errors.
- key_not_valid: The API key is missing or invalid.
- missing parameters: Insufficient validations left for this request.
A fail response may indicate various issues, such as non-existent addresses, full mailboxes, use of disposable email services, invalid format, role accounts, lacking DNS or MX server records, or listings in your internal or domain blacklists.
Crafting an Email Validation Regex in JavaScript
Creating an effective email validation regex pattern in JavaScript requires careful consideration. Here's an example of a simple email regex pattern:
const emailRegex = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$/;
Key components of the regex pattern:
^
: Start of the string.[a-zA-Z0-9._-]+
: Match one or more alphanumeric characters, dots, underscores, or hyphens for the username part.@
: Match the "@" symbol.[a-zA-Z0-9.-]+
: Match one or more alphanumeric characters, dots, or hyphens for the domain name.\.
: Match a period (dot), which separates the domain name and top-level domain (TLD).[a-zA-Z]{2,4}
: Match the TLD, consisting of 2 to 4 alphabetical characters.$
: End of the string.
Common Issues in JavaScript Email Validation
While crafting email validation regex patterns in JavaScript, developers often encounter common issues:
Overly Restrictive Patterns: Some regex patterns might be too strict, rejecting valid email addresses.
Incomplete Validation: Basic regex patterns only verify the email format, not the existence of the email address.
Complexity: Crafting a perfect regex pattern that covers all edge cases can be challenging and complex.
Best Practices for JavaScript Email Validation
To ensure effective email validation in JavaScript, consider these best practices:
Use Existing Patterns: Utilize well-tested and widely accepted regex patterns for email validation.
Backend Validation: Complement regex validation with backend validation to check email existence and minimize false positives.
Informative Error Messages: Provide clear and informative error messages to guide users when their input is invalid.
Regular Updates: Keep regex patterns up-to-date to accommodate evolving email formats.
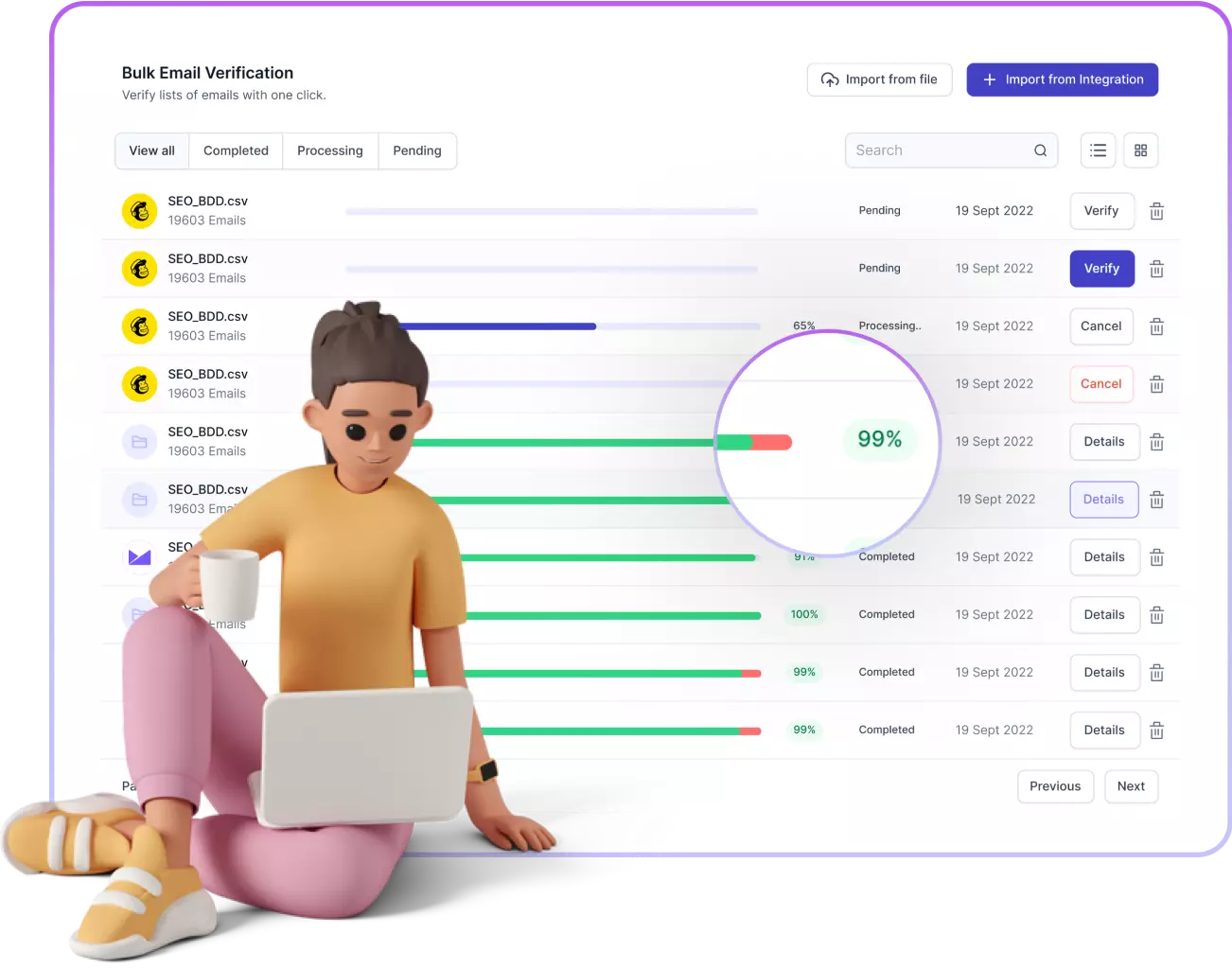
Adding to the capabilities of email validation in JavaScript, the 'Email List Validation Bulk Verification' feature is a game-changer for handling large volumes of email addresses efficiently. This functionality is particularly useful when you need to validate extensive lists of email addresses, such as in marketing campaigns or user database cleanups. The bulk verification process quickly scans and validates each email address in your list against a comprehensive set of criteria, including format correctness, domain existence, and overall deliverability. This ensures that your email campaigns reach their intended recipients and significantly reduces bounce rates. The bulk verification process is streamlined and automated, making it easy to integrate into your existing workflows. By using the Email List Validation Bulk Verification feature, you can maintain the integrity of your email lists, enhance campaign effectiveness, and save valuable time and resources.
Frequently Asked Questions (FAQs)
Let's address some frequently asked questions about email validation in JavaScript using regular expressions:
Q1: Can regex validate all email addresses accurately?
Regex can validate email format, but it cannot guarantee the existence of the email address. Combining regex with backend validation is recommended for comprehensive validation.
Q2: What is the most widely accepted regex pattern for email validation in JavaScript?
A common and widely accepted regex pattern for email validation in JavaScript is /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$/
.
Q3: Are there regex patterns for international email addresses?
Yes, regex patterns that accommodate international email addresses exist, but they tend to be more complex due to the diversity of character sets.
Q4: How can I prevent overly restrictive regex patterns?
To prevent overly restrictive patterns, use well-established regex patterns and consider allowing a wide range of valid characters.
Q5: Should I update my regex patterns regularly?
Yes, keeping regex patterns up-to-date is essential to accommodate new email formats and prevent false negatives.
Conclusion
In conclusion, mastering email validation in JavaScript using regular expressions is essential for web developers. By understanding its significance, crafting regex patterns, and applying best practices, you can ensure that your web forms collect accurate and valid email addresses. Remember to complement regex validation with backend validation for a more comprehensive approach, and keep your patterns up-to-date to adapt to evolving email formats. Elevate your web development skills with foolproof email validation today!
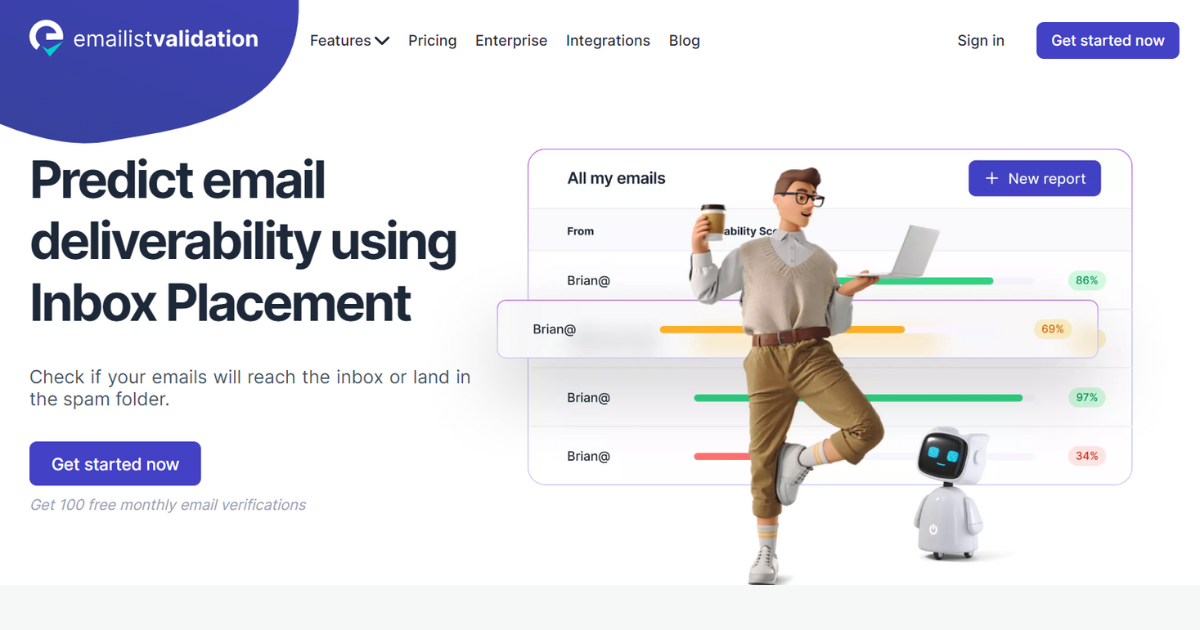
Discover unbeatable value with our flexible pricing plans tailored for your email verification needs. Whether you're a startup or a large enterprise, our Pay-As-You-Go and subscription options are designed to fit any budget and scale. Start small with 2,500 emails for just $19, and experience our service without a hefty initial investment. Or, choose from our monthly plans to get more emails at lower rates, perfect for businesses with ongoing verification needs. Our tiered pricing ensures you get the most cost-effective rate, dropping as low as $0.000495 per email for our bulk packages.
Ready for a serious commitment? Our annual plans offer substantial savings for a year's worth of peace of mind. And for high-volume enterprises, we provide customized solutions over 10 million emails. Contact us to discuss how we can meet your specific requirements.
Don't miss out on the opportunity to enhance your email marketing strategy with our reliable verification services. Visit our pricing page for more details, and get started with a plan that's right for you. Your journey towards cleaner lists and better engagement starts here!