Email validation is a critical step in building robust applications that require accurate user data. One way to achieve this is by using the Zod library, a TypeScript-first schema validation library that provides a simple and powerful way to validate email addresses. In this article, we will explore what email validation is, why it is important, and how you can use Zod to improve your email validation process.
What is Email Validation?
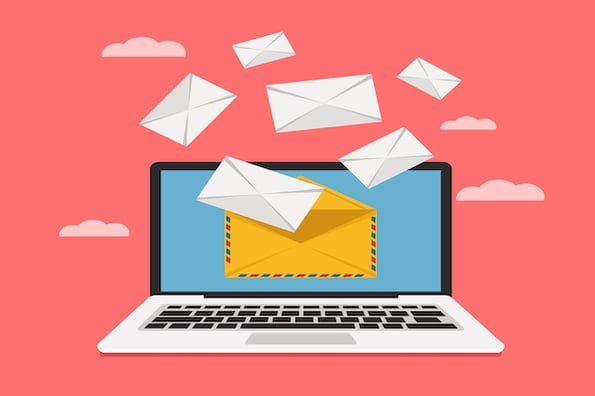
Email validation is the process of verifying if an email address is valid and exists. This process involves checking the syntax, domain, and mailbox of an email address to ensure that it is properly formatted and can receive emails. Email validation is essential in preventing invalid or fake email addresses from entering your system, ensuring that your user data is accurate and reliable.
Why is Email Validation Important?
Email validation is critical in maintaining the integrity of your user data. Invalid or fake email addresses can lead to bounced emails, undelivered messages, and potential security risks. By validating email addresses, you can ensure that your system only contains accurate and verified email addresses, which can save you time, resources, and help prevent fraud or spam. Additionally, email validation can improve your user experience by preventing typos or incorrect email addresses from being submitted, which can reduce user frustration and increase conversions.
How to Perform Email Validation with Zod
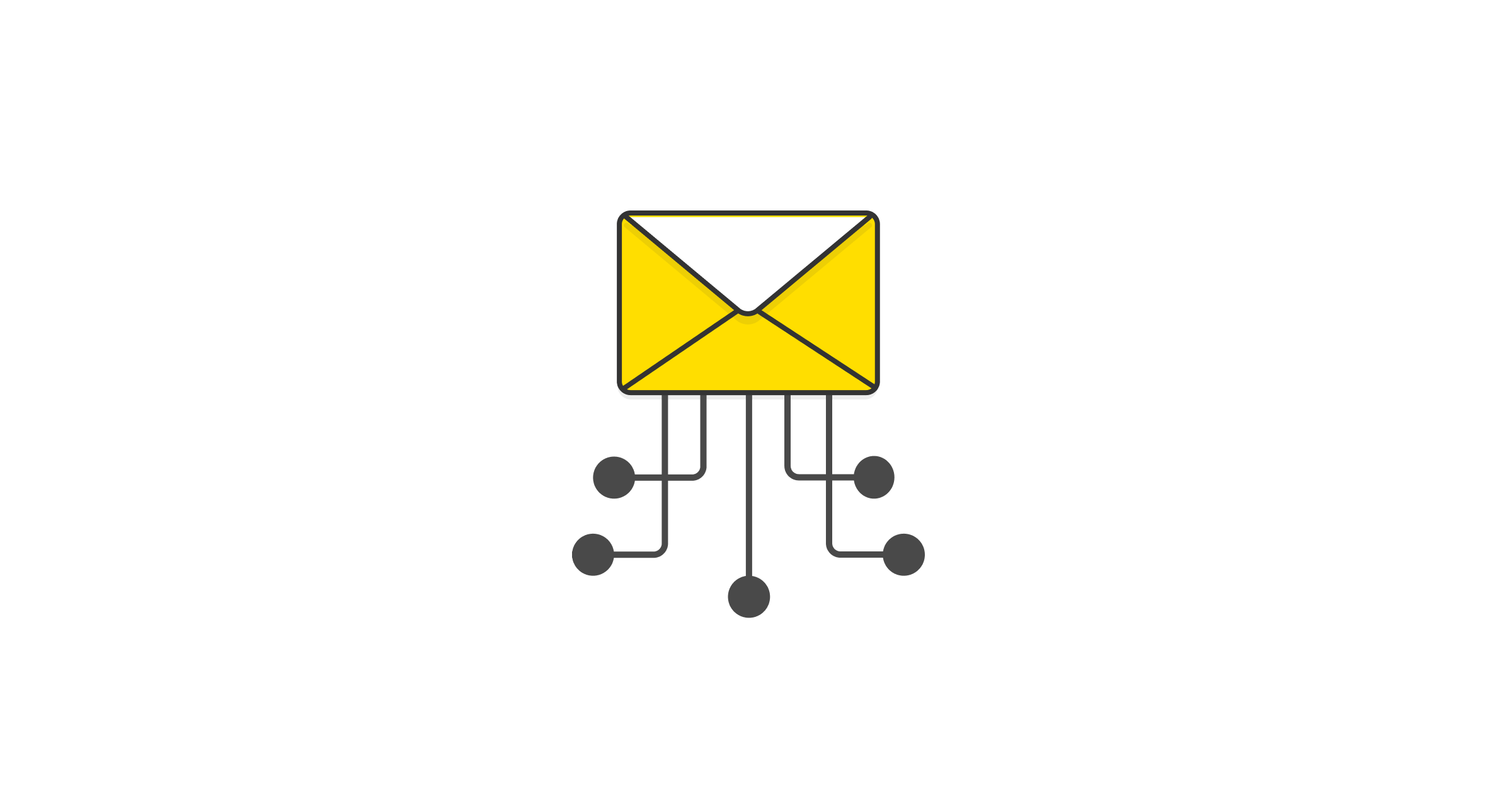
Zod is a TypeScript-first schema validation library that provides a simple and intuitive way to validate email addresses. To use Zod for email validation, you will need to install the Zod library and import it into your project. Once you have done that, you can create a Zod schema that defines the shape and type of your email address. Here is an example of how to create a Zod schema for email validation:
import { z } from 'zod';const emailSchema = z.string().email();
The code above creates a Zod schema that defines a string value with an email format. This means that any string that is not properly formatted as an email address will be rejected by the schema. You can then use this schema to validate email addresses in your application. Here is an example of how to use the Zod schema to validate an email address:
const email = '[email protected]';try { emailSchema.parse(email); console.log('Email is valid'); } catch (error) { console.log('Email is invalid'); }
The code above creates an email variable with a valid email address and then tries to parse it using the Zod schema. If the email address is valid, the code will log 'Email is valid', otherwise, it will log 'Email is invalid'. By using Zod, you can simplify your email validation process and ensure that your user data is accurate and reliable.
Conclusion
Email validation is a critical step in building robust applications that require accurate user data. By using the Zod library, you can simplify your email validation process and improve the integrity of your user data. Zod provides a simple and intuitive way to define and validate email addresses, which can save you time, resources, and help prevent fraud or spam. If you are building an application that requires email validation, consider using Zod to simplify your code and improve your data integrity.