When it comes to email validation in JavaScript, regular expressions are often the go-to solution. However, if you prefer a regex-free approach or want to explore alternative methods, this article is for you. We'll discuss various techniques and strategies to validate email addresses without relying on regular expressions, ensuring accurate and reliable validation.
The Limitations of Regex for Email Validation
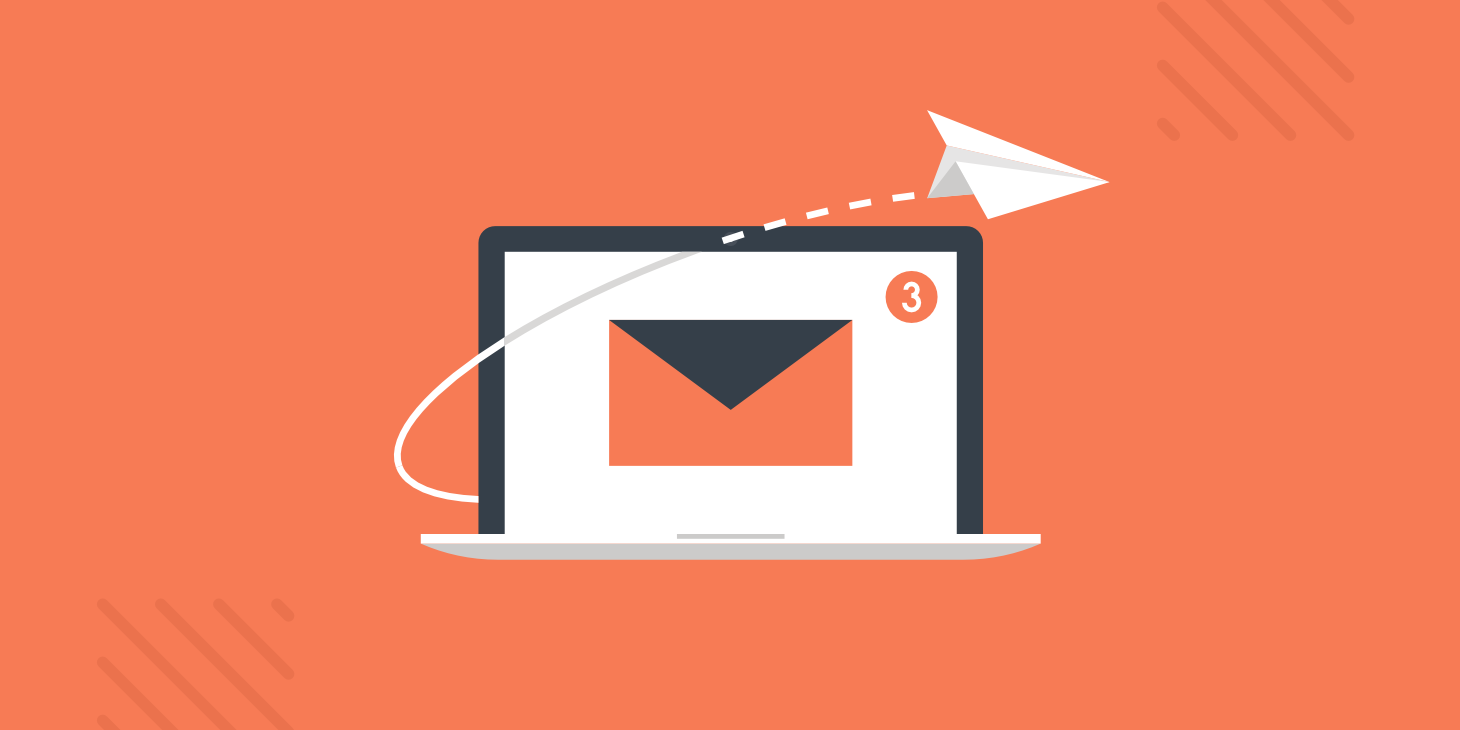
Although regular expressions are widely used for email validation, they can be complex and error-prone. They often struggle to cover all possible email address variations and can result in false positives or false negatives. Additionally, regex patterns can be difficult to maintain and understand for developers who are not familiar with them.
Alternative Approaches for Email Validation
1. Syntax Validation: One way to validate email addresses is by checking their syntax. JavaScript provides built-in methods like 'indexOf', 'lastIndexOf', and 'split' that can be used to detect specific characters or patterns in an email address. For example, you can check if the email address contains an '@' symbol and if it has a valid domain extension.
2. DNS Lookup: Another approach is to perform a DNS lookup on the domain part of the email address. By querying the DNS server, you can determine if the domain exists and if it has a valid mail exchanger (MX) record. This method ensures that the email address corresponds to a valid and active domain.
3. SMTP Verification: SMTP (Simple Mail Transfer Protocol) verification involves establishing a connection with the recipient's mail server and simulating the sending of an email. If the server responds positively, it indicates that the email address is valid and can receive messages. However, this method requires server-side programming and is not suitable for client-side email validation.
Implementing Email Validation without Regex in JavaScript
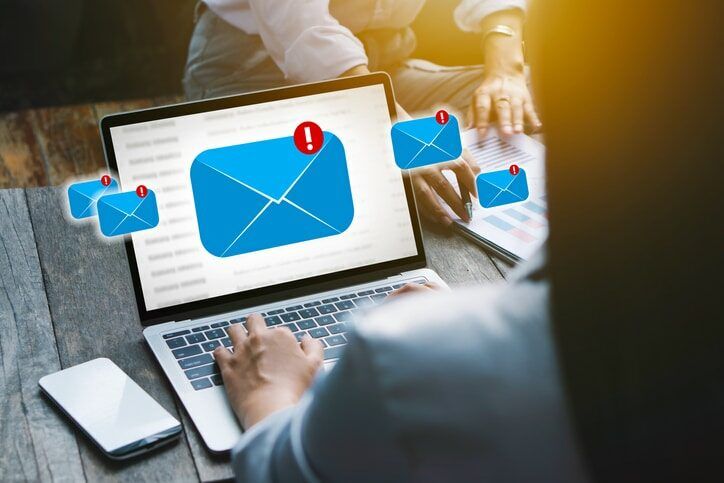
Now, let's dive into the implementation details of email validation without regex in JavaScript:
Syntax Validation Example:
function validateEmailSyntax(email) {\n var atIndex = email.indexOf('@');\n var dotIndex = email.lastIndexOf('.');\n return (atIndex > 0 && dotIndex > atIndex);\n}\n\nvar email = '[email protected]';\nvar isValid = validateEmailSyntax(email);\nconsole.log(isValid); // Output: true\n
DNS Lookup Example:
function validateEmailDomain(email) {\n var domain = email.split('@')[1];\n // Perform DNS lookup and validate domain\n // ... (DNS lookup logic)\n return isValid;\n}\n\nvar email = '[email protected]';\nvar isValid = validateEmailDomain(email);\nconsole.log(isValid); // Output: true\n
Conclusion
Validating email addresses in JavaScript without relying on regular expressions is possible by leveraging alternative approaches such as syntax validation, DNS lookup, and SMTP verification. These methods provide more flexibility, accuracy, and maintainability compared to regex-based validation.