As an expert in web development, one of the most important aspects of creating a website is ensuring that the user input is accurate and valid. When it comes to collecting email addresses, there are a few different ways to approach validation. In this article, we will explore the importance of validating the email field, the different methods for doing so, and answer some commonly asked questions about this topic.
Why Validate the Email Field?
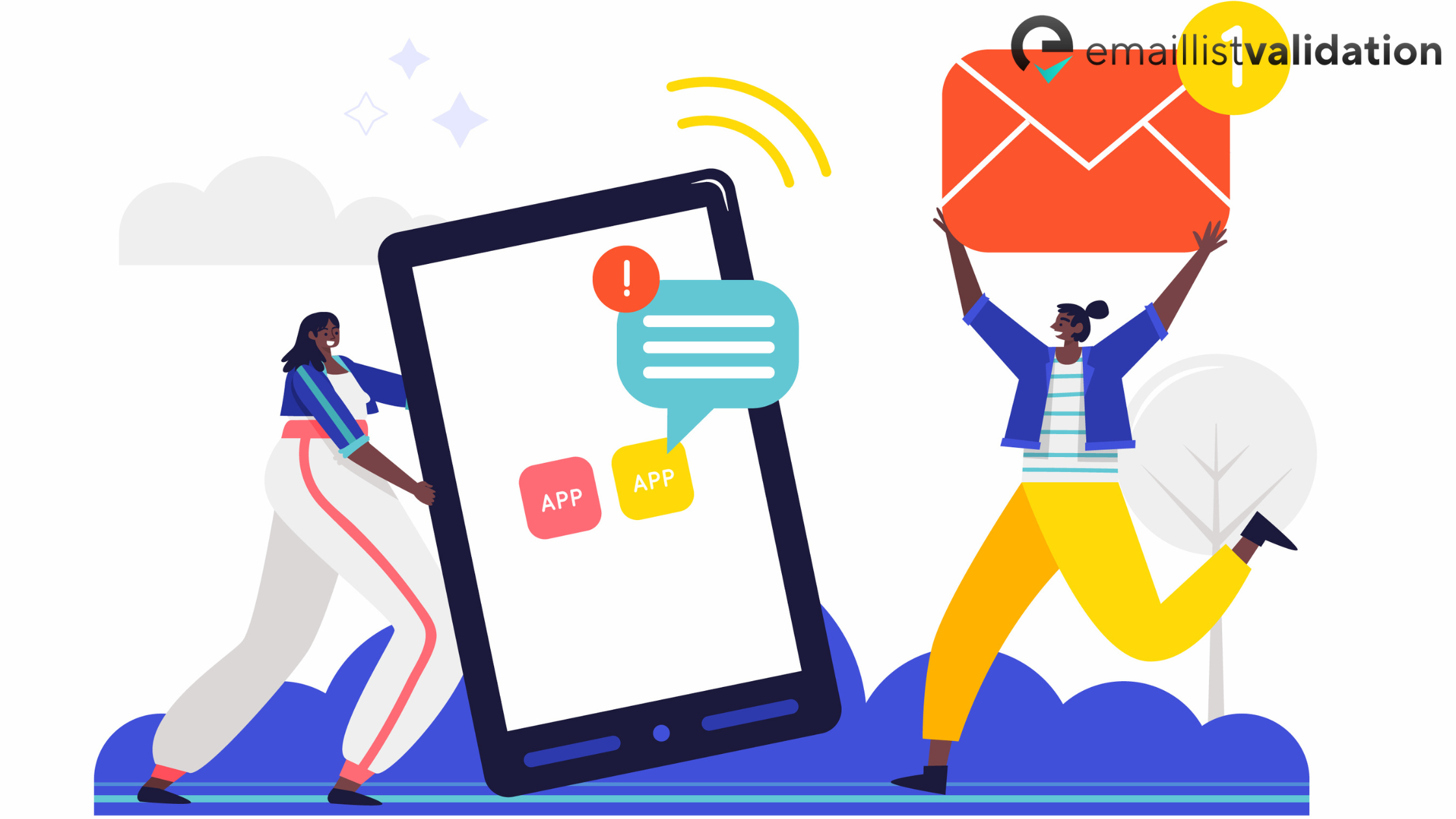
First things first, why is it so important to validate the email field? The answer is simple: accuracy. Collecting email addresses that are not valid can lead to a variety of problems, such as:
- Sending emails to non-existent email addresses, which can hurt your email deliverability rate and potentially get your domain marked as spam.
- Allowing spam bots to submit fake email addresses, which can lead to an increase in spam complaints from real email recipients.
- Not being able to contact users who have given you their email address, which defeats the purpose of collecting it in the first place.
By validating the email field, you can ensure that the email addresses you collect are accurate and valid, which will ultimately lead to better communication with your users and a better user experience overall.
Methods for Validating the Email Field
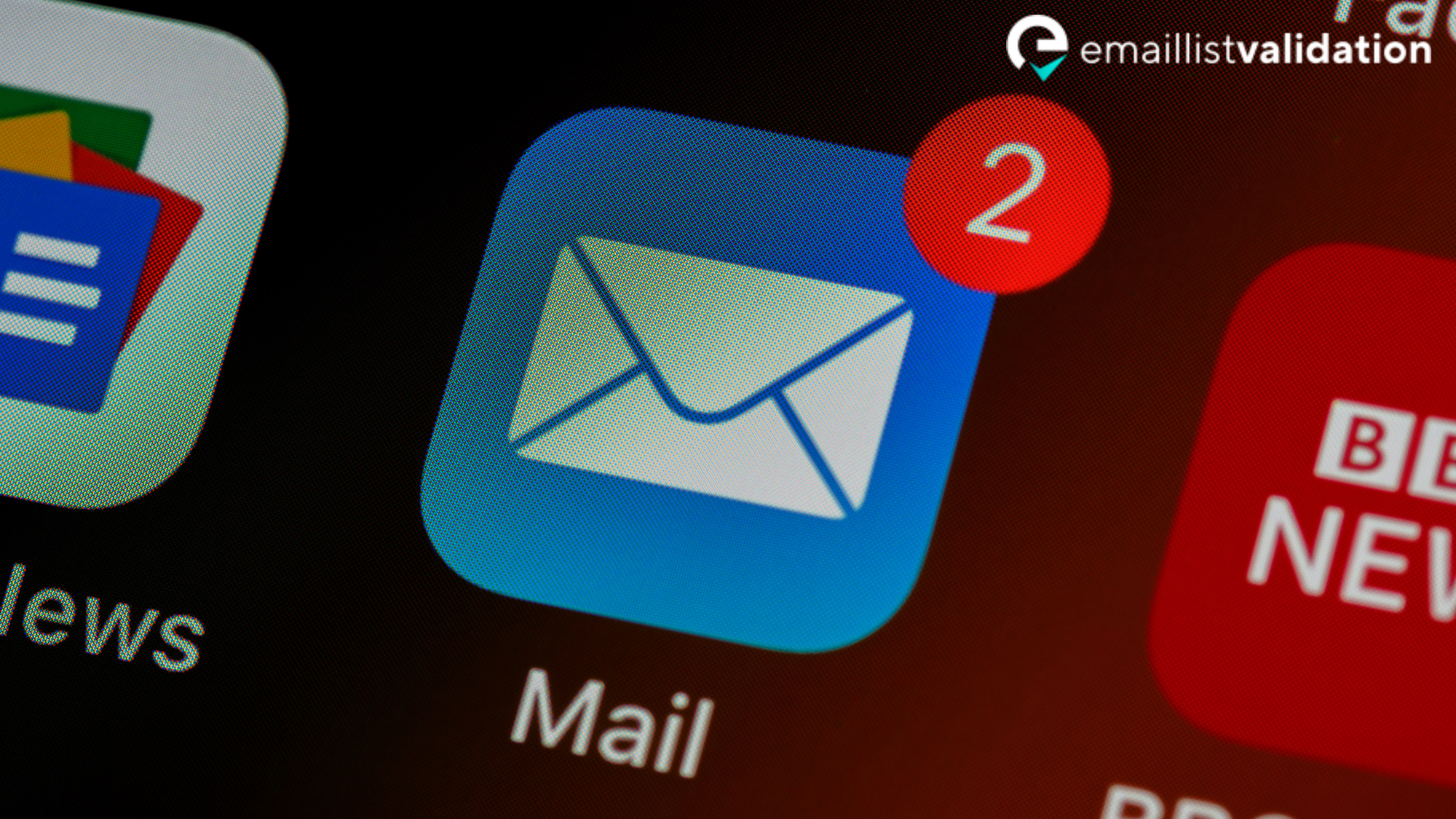
There are a few different methods for validating the email field, each with their own pros and cons. Let's take a closer look at some of the most popular methods:
HTML5 Validation
One of the easiest ways to validate the email field is to use HTML5 validation. This is done by simply adding the ""type"" attribute ""email"" to your input element, like so:
<
This will automatically validate the email address and display an error message if the format is incorrect. The benefit of this method is that it requires no additional JavaScript, but the downside is that it only checks for basic format errors and does not check if the email address actually exists.
JavaScript Validation
If you want more advanced validation, you can use JavaScript to check if the email address is valid and exists. Here is an example of how to do this:
function validateEmail(email) {
var re = /\S+@\S+.\S+/
return re.test(email)
}
This function checks if the email address is in a valid format using a regular expression. To check if the email address actually exists, you can use an API like Verifalia (more on that later).
Third-Party Validation
If you don't want to write your own validation code, you can use a third-party service like Verifalia to validate email addresses for you. Verifalia checks if the email address is valid and actually exists, and returns a result that you can use in your own code. Here is an example of how to use Verifalia:
Verifalia.verify(email).then(function(result) {
if (result.status === ""valid"") {
// email is valid, do something
} else {
// email is not valid, do something else
}
})
This method requires some additional setup and potentially a cost, but it provides the most accurate validation results.
Commonly Asked Questions
What is the best method for validating the email field?
The best method for validating the email field depends on your specific needs. If you only need basic format validation, HTML5 validation is a good option. If you need more advanced validation, JavaScript or third-party validation is recommended.
What is the most accurate way to validate an email address?
The most accurate way to validate an email address is to use a third-party service like Verifalia, which checks if the email address is valid and actually exists.
What are some common email address format errors?
Some common email address format errors include missing the ""@"" symbol, using invalid characters in the email address, and not including a top-level domain like "".com"" or "".org"".
Can I validate the email field without using JavaScript?
Yes, you can use HTML5 validation to validate the email field without using JavaScript. However, this only checks for basic format errors and does not check if the email address actually exists.
Is it necessary to validate the email field?
Yes, it is necessary to validate the email field to ensure accuracy and prevent issues like sending emails to non-existent email addresses or allowing spam bots to submit fake email addresses.
Conclusion
Validating the email field is an important aspect of web development that should not be overlooked. By ensuring that the email addresses you collect are accurate and valid, you can improve communication with your users and provide a better user experience overall. Whether you choose to use HTML5 validation, JavaScript validation, or a third-party service like Verifalia, make sure to take the time to validate your email field and prevent potential issues down the line.